第01期 - 新的开始
这里是 Chiloh 记录日常观察的地方,每周日发布,感谢你的阅读。
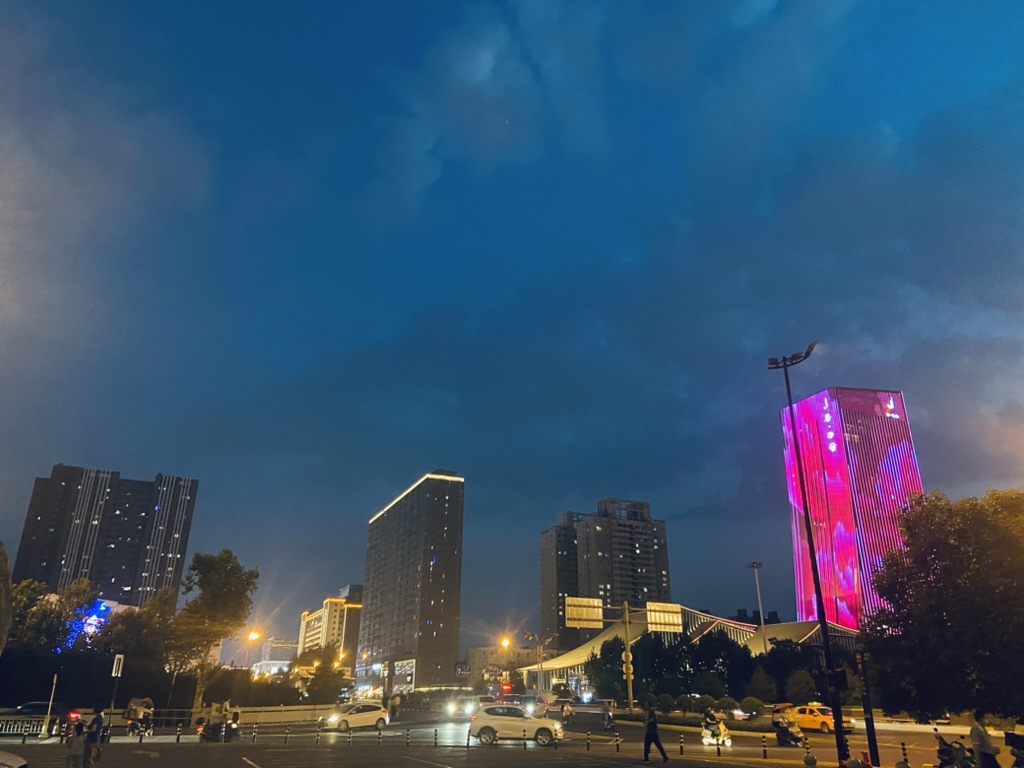
封面图拍摄于22年的下班路上,也是我微信朋友圈的背景图。很喜欢这种能闻到城市烟火气的照片,让人觉得宁静的生活一切都很好。
一直以来,都想写一个自己的周刊,分享自己的观察与见闻。直到现在,才正式开始。本篇是第一篇,就如同生活一样,我定义它为:新的开始。
文章
视频
感受
这周对于 ChatGPT 有了全新的感受,其可以轻松帮助一个非专业领域的人,创造出一个完整的作品出来。
案例:作为一个不懂代码开发的普通人,想要实现一个功能:在本地浏览器给图片加水印,水印可以调整颜色,透明度和倾斜角度,可以实时预览。
源代码:
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>图片水印工具</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
#imageCanvas {
border: 1px solid #ddd;
max-width: 500px; /* 最大宽度限制 */
max-height: 500px; /* 最大高度限制 */
}
div {
margin-top: 20px;
}
</style>
</head>
<body>
<h2>图片水印工具</h2>
<input type="file" id="imageInput" accept="image/*" />
<br>
<canvas id="imageCanvas"></canvas>
<br>
<div>
<label>水印文字: <input type="text" id="watermarkText" /></label>
<label>颜色: <input type="color" id="watermarkColor" /></label>
<label>透明度: <input type="range" id="watermarkOpacity" min="0" max="1" step="0.1" value="1" /></label>
<label>倾斜角度: <input type="range" id="watermarkAngle" min="0" max="360" value="0" /></label>
<button id="applyWatermark">应用水印</button>
</div>
<script>
const imageInput = document.getElementById('imageInput');
const imageCanvas = document.getElementById('imageCanvas');
const ctx = imageCanvas.getContext('2d');
let uploadedImage = null;
// 当选择图片时进行处理
imageInput.addEventListener('change', function (e) {
const file = e.target.files[0];
const reader = new FileReader();
reader.onload = function (event) {
const img = new Image();
img.onload = function () {
const scale = Math.min(500 / img.width, 500 / img.height);
const width = img.width * scale;
const height = img.height * scale;
imageCanvas.width = width;
imageCanvas.height = height;
ctx.drawImage(img, 0, 0, width, height);
uploadedImage = img;
}
img.src = event.target.result;
}
reader.readAsDataURL(file);
});
// 应用水印
document.getElementById('applyWatermark').addEventListener('click', function () {
if (!uploadedImage) return;
ctx.clearRect(0, 0, imageCanvas.width, imageCanvas.height);
const scale = Math.min(500 / uploadedImage.width, 500 / uploadedImage.height);
const width = uploadedImage.width * scale;
const height = uploadedImage.height * scale;
ctx.drawImage(uploadedImage, 0, 0, width, height);
const text = document.getElementById('watermarkText').value;
const color = document.getElementById('watermarkColor').value;
const opacity = document.getElementById('watermarkOpacity').value;
const angle = document.getElementById('watermarkAngle').value;
ctx.save();
ctx.globalAlpha = opacity;
ctx.fillStyle = color;
ctx.font = "30px Arial";
ctx.translate(imageCanvas.width / 2, imageCanvas.height / 2);
ctx.rotate(angle * Math.PI / 180);
// 绘制多行水印
const lineHeight = 40; // 行高
const lines = text.split('\n'); // 根据换行符分割文本
for (let i = 0; i < lines.length; i++) {
ctx.fillText(lines[i], -ctx.measureText(lines[i]).width / 2, i * lineHeight);
}
ctx.restore();
});
</script>
</body>
</html>